We can't find the internet
Attempting to reconnect
Something went wrong!
Hang in there while we get back on track
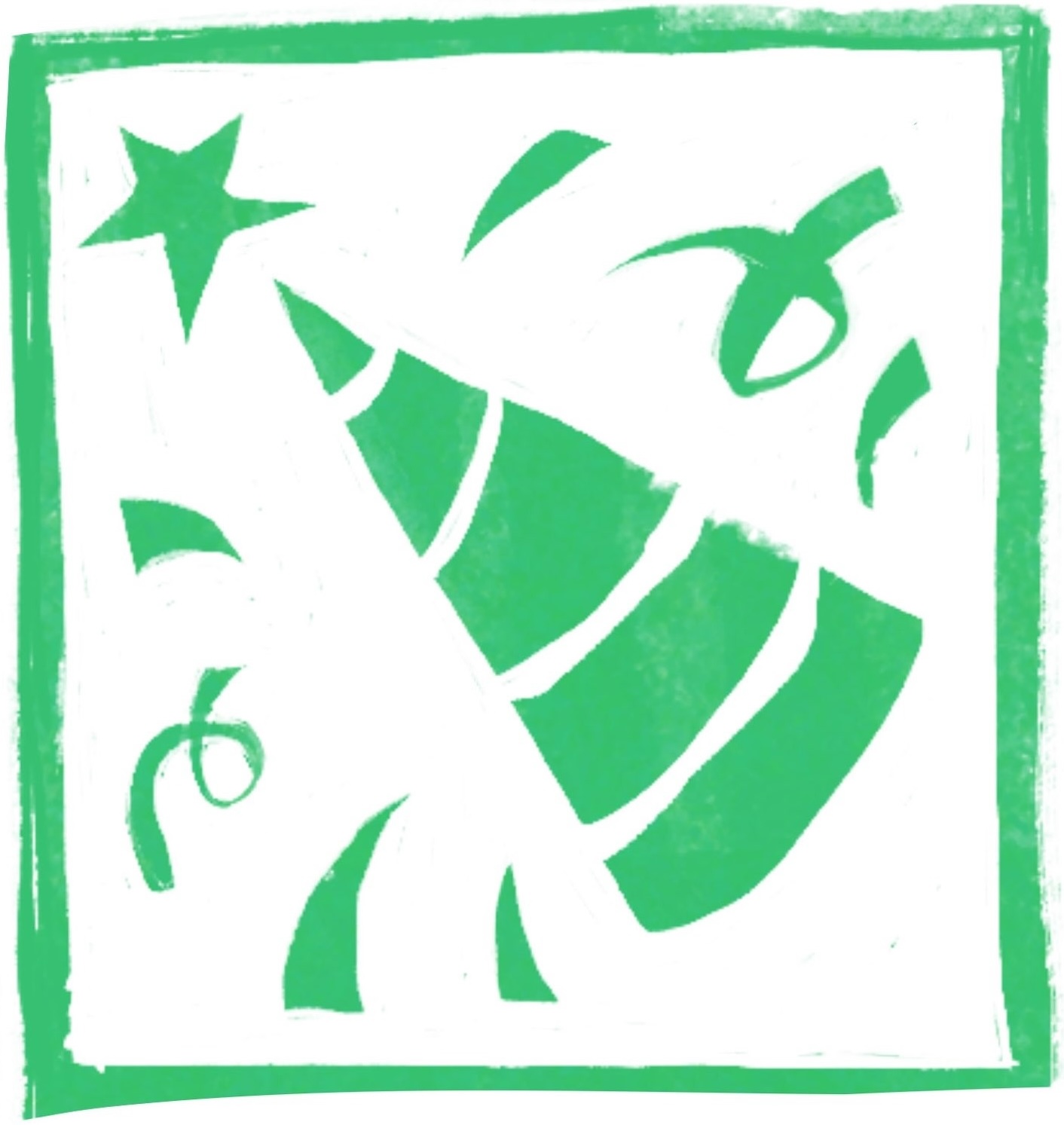
Host a party from the command line
Use the Shindig REST API to host a party, keep track of your guest list, and send out reminders. No login required.
Making a Partiful is like sending your mother a Hallmark card. Why not craft the invitations yourself? Shindig is like an easel and paint kit to make your own party website.
Parties made with Shindig (so far):
Get started
1. Create party
Create a party with a POST request to https://shindig.lol/api/v0/parties
. For example:
curl https://shindig.lol/api/v0/parties -X POST
You’ll get back a response with the party’s slug and an auth token. Save both these values. The slug is a unique identifier for the party; you’ll use it to reference the party in future API requests. The auth token gives you admin permissions.
Example response:
{
"data": {
"message": "Success! New party created. Don't forget to save your auth token — you'll only see it once and it gives you full access to your party's guest list.",
"auth_token": "RmPC0g0DDCHLlLxjM9R_4JvV7a7oOTRMMvT7Fn6KynQ",
"slug": "partial-star-shindig"
}
}
You can also provide your own slug:
curl https://shindig.lol/api/v0/parties -X POST -H "Content-Type: application/json" -d '{"party": {"slug": "my-unique-party-identifier"}}'
2. Invite guests
To create a guest for your party, make a post request to /parties/:slug/guests
with a guest payload:
curl 'https://shindig.lol/api/v0/parties/phony-spread-fiesta/guests' \
--header 'Content-Type: application/json' \
--data '{"guest": {"name": "Dice", "status": "yes", "phone_number": "16467611318"}}'
Name and status are required; status can take values “yes”, “no”, and “maybe”. Phone number is optional.
To make this process easier for guests, you can deploy your own frontend (docs coming soon).
3. Customize
What makes Shindig special is that your invitations can be completely unique to your party.
To collect additional information from your guests, use the “public” and “private” fields:
-
public: contains arbitrary public information. For example,
{"comment": "Hi everyone! Can't wait."}
-
private: contains arbitrary private information. For example,
{"allergies": "peanuts"}
Here’s another example of a guest creation request using all possible fields:
curl 'https://shindig.lol/api/v0/parties/phony-spread-fiesta/guests' \
--header 'Content-Type: application/json' \
--data '{"guest": {"name": "Dice", "phone_number": "16467611318", "private": {"allergens": "peanuts"}, "public": {"comment": "can'\''t wait!"}}}'
4. Message guests
To send an SMS message to your guest list, POST to /parties/:slug/message
.
You’ll need to include your auth token like so:
curl https://shindig.lol/api/v0/parties/:slug/messages
-X POST -H "Authorization: Bearer <auth-token> -d '{"message": "See you tomorrow!"}'
API reference
Host endpoints
Create party
POST request to /api/v0/parties
.
Optionally, provide a slug:
curl https://shindig.lol/api/v0/parties -X POST -H "Content-Type: application/json" -d '{"party": {"slug": "2024-01-01-wine-and-cheese"}}'`
List guests
GET request to /parties/:slug/guests
. If you provide an auth token, it includes guest phone numbers and private metadata.
curl https://shindig.lol/api/v0/parties/:slug/guests -H "Authorization: Bearer <auth-token>"
Example output:
[
{"name": <name>, "status": <status>, "phone_number": <number>, "public": <object>, "private": <object>}
...
]
Create message
POST to /parties/:slug/message
to send an SMS message to your guest list.
curl https://shindig.lol/api/v0/parties/:slug/messages -X POST -H "Authorization: Bearer <auth-token> -d '{"message": "See you tomorrow!"}'
This will send an SMS message to all guests who have a phone number set.
Get party
GET request to /parties
. (This will mostly be useful if you forget your party’s slug and need to recover it.)
curl https://shindig.lol/api/v0/parties -H "Authorization: Bearer <auth-token>
Guest endpoints
Create guest (RSVP)
POST to /parties/:slug/guests
:
curl 'https://shindig.lol/api/v0/parties/phony-spread-fiesta/guests' \
--header 'Content-Type: application/json' \
--data '{"guest": {"name": "Dice", "status": "yes", "phone_number": "16467611318"}}'
Required:
- name (string)
- status (“yes”, “no”, or “maybe”)
Optional:
- phone_number (some accepted formats: “+15555555” or “+1 555-5555”)
- public (json object, for example {“comment”: “Hi everyone! Can’t wait.”})
- private (json object, for example {“allergies”: “peanuts”})
Example of a guest POST request with all possible fields:
curl 'https://shindig.lol/api/v0/parties/phony-spread-fiesta/guests' \
---header 'Content-Type: application/json' \
---data '{"guest": {"name": "Dice", "status": "maybe", "phone_number": "16467611318", "private": {"allergens": "peanuts"}, "public": {"comment": "literally cannot wait"}}}'
List guests
GET request to /parties/:slug/guests
.
curl https://shindig.lol/api/v0/parties/:slug/guests
Example output:
[
{"name": <name>, "status": <status>, "public": <object>, "private": <object>}
...
]
To view “number” and “private” as well, provide an auth token.